Tags: crypto twotimepad
Rating:
# Crypto: TwoTimePad
> Author: cogsworth64
>
> Description: One-time pads are perfectly information-theoretically secure, so I should be safe, right?
[chall.py](https://github.com/nopedawn/CTF/blob/main/WolvCTF24/Beginner-TwoTimePad/chall.py)
[eFlag.bmp](https://github.com/nopedawn/CTF/blob/main/WolvCTF24/Beginner-TwoTimePad/eFlag.bmp)
[eWolverine.bmp](https://github.com/nopedawn/CTF/blob/main/WolvCTF24/Beginner-TwoTimePad/eWolverine.bmp)
Second challenge crypto beginner is a classic example of the misuse a one-time pad (OTP). Called `TwoTimePad` is a hint towards this. The key should be random, secret, and never reused. However in this challenge, the same key is used to encrypt two different encrypted files (`eWolverine.bmp` & `eFlag.bmp`).
```python
from Crypto.Random import random, get_random_bytes
BLOCK_SIZE = 16
with(open('./genFiles/wolverine.bmp', 'rb')) as f:
wolverine = f.read()
with(open('./genFiles/flag.bmp', 'rb')) as f:
flag = f.read()
w = open('eWolverine.bmp', 'wb')
f = open('eFlag.bmp', 'wb')
f.write(flag[:55])
w.write(wolverine[:55])
for i in range(55, len(wolverine), BLOCK_SIZE):
KEY = get_random_bytes(BLOCK_SIZE)
w.write(bytes(a^b for a, b in zip(wolverine[i:i+BLOCK_SIZE], KEY)))
f.write(bytes(a^b for a, b in zip(flag[i:i+BLOCK_SIZE], KEY)))
```
The headers of the BMP files contain important information such as the size, color depth, compression method, etc. In the provided Python script, the headers of the original images are preserved. However, if we XOR the two encrypted images together, the headers are also XOR-ed.
Then, we need to XOR the two encrypted images together. Since the same key was used for both, this will effectively cancel out the key, leaving with the XOR of the two original images.
```python
HEADER_SIZE = 55 # Size of the BMP header
with open('eWolverine.bmp', 'rb') as f:
eWolverine = f.read()
with open('eFlag.bmp', 'rb') as f:
eFlag = f.read()
header = eWolverine[:HEADER_SIZE]
xor = header + bytes(a^b for a, b in zip(eWolverine[HEADER_SIZE:], eFlag[HEADER_SIZE:]))
with open('out.bmp', 'wb') as f:
f.write(xor)
```
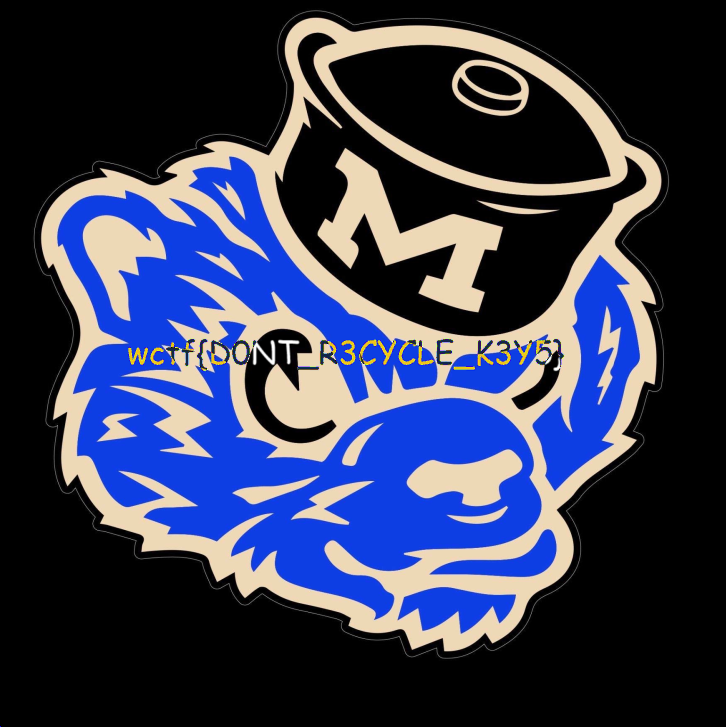
> Flag: `wctf{D0NT_R3CYCLE_K3Y5}`